最短路径
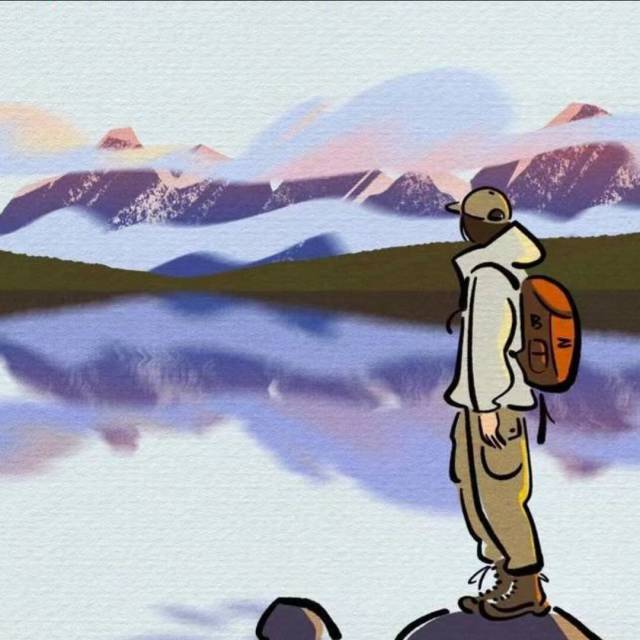
n:点数
m:边数
稠密图:
相对来说线比点多
显然易见,稠密图的路径太多了,所以就用点来找,也就是抓重点;
稀疏图:
相对来说点比线多
点很多,但是连线不是很多的时候,对应的就是稀疏图,稀疏图的路径不多,所以按照连接路径找最短路,这个过程运用优先队列,能确保每一次查找保留到更新到队列里的都是最小的,同时还解决了两个点多条路选择最短路的问题;
Dijkstra算法求解最短路
基于贪心算法
朴素Dijkstra算法
dis[i]=INF dis[1]=0
2.(s : 当前已确定最短距离的)
For i:0~n (循环n次可得n个结点的最短距离)
t <- 不在s 中的距离最近的点
s <- t
用t更新其他点的距离
更新方式: 判断l->x与l->t->x
dis[x] > dis[t] + w
例题链接:849. Dijkstra求最短路 I - AcWing题库
1 |
|
堆优化版Dijkstra
堆:手写堆或者优先队列——使用优先队列
例题链接:850. Dijkstra求最短路 II - AcWing题库
1 |
|
待更新……
- 标题: 最短路径
- 作者: Cealivanus Kwan
- 创建于 : 2024-10-14 22:06:59
- 更新于 : 2024-12-19 22:29:32
- 链接: https://redefine.ohevan.com/2024/10/14/最短路径/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。